4. Example Python
I have created a Python example in a Notebook in Microsoft Fabric. But it could be done anywhere.
The result is the bearer (just to show you) and the companies. It looks like this:
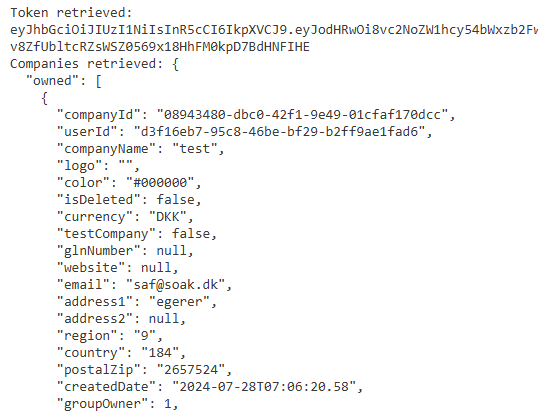
You just have to put in the username and api-key, and then you are good to go.
IMPORTANT!!!! You mig need to install request or json.
import requests
import json
# Define the URLs
token_url = "https://api.ubidogy.com/api/getToken"
companies_url = "https://api.ubidogy.com/api/v1/Company/GetCompanies"
# Define the payload for token request
token_payload = {
"username": "<YOUR USERNAME>",
"apiKey": "<YOUR API KEY>"
}
# Function to get bearer token
def get_bearer_token(url, payload):
response = requests.post(url, json=payload)
response.raise_for_status() # Raise an error for bad status codes
token = response.json().get("token")
return token
# Function to get companies
def get_companies(url, token):
headers = {
"Authorization": f"Bearer {token}"
}
response = requests.get(url, headers=headers)
response.raise_for_status() # Raise an error for bad status codes
companies = response.json()
return companies
# Get the bearer token
try:
token = get_bearer_token(token_url, token_payload)
print("Token retrieved:", token)
except requests.RequestException as e:
print("Error fetching token:", e)
# Get the companies
try:
companies = get_companies(companies_url, token)
print("Companies retrieved:", json.dumps(companies, indent=2))
except requests.RequestException as e:
print("Error fetching companies:", e)
0 Comments